Embed Glide Deposit
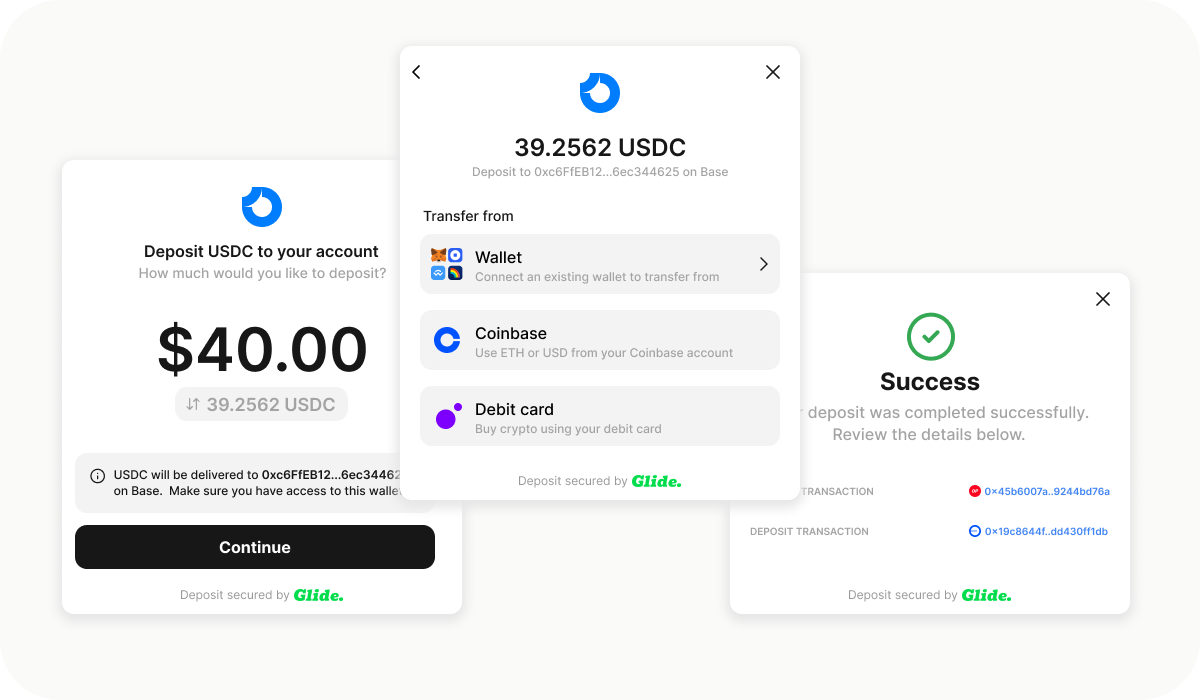
Let users deposit tokens into your app using Glide's pre-built React components. You can customize the components to match your app's design and branding.
Setup
Install the Glide React SDK
npm install @paywithglide/glide-react
The Hook
The Glide React SDK comes with a useGlideDeposit
hook that you can use to open the deposit modal.
const { openGlideDeposit } = useGlideDeposit({
/* Your App ID, provided by the Glide Team */
app: "my-app",
/* The wallet address that will receive the deposit.
* This is usually the user's connected wallet addres.
*/
recipient: "0x0...",
});
Open the Deposit Modal
You can now call the openGlideDeposit
function to open the deposit modal.
<button onClick={() => openGlideDeposit()}>Deposit now</button>
That's it!
You can now monitor the user's wallet address for balance updates.
Optional - Wallet Provider
If users have already connected a wallet in your app, you can pass the wallet provider to the useGlideDeposit
hook. This will allow users to deposit from their connected wallet.
const { openGlideDeposit } = useGlideDeposit({
...
/* The walletProvider object acts as a bridge between your app
* and the embedded pay modal.
* This bridge is used to send transactions to the user's
* connected wallet, along with other wallet-related operations.
*/
walletProvider: {
/* A list of chain ids that the user's connected wallet supports */
availableChainIds: [1, ...],
/* The current chain id that the user's connected wallet is on */
currentChainId: 1,
/* The user's connected wallet address */
address: "0x0...",
switchChainAsync: async ({chainId}) => {
// Switch the user's connected wallet to the new chain
},
sendTransactionAsync: async (tx) => {
// Send the transaction to the user's connected wallet
// Return the transaction hash
},
},
});
Optional - Gasless transactions
If you want the user to be able to transfer certain tokens without having to hold gas, you can enable gasless transactions. Many tokens such as USDC support this.
All you have to do is update your walletProvider
and implement the signTypedDataAsync
function to handle gasless transactions. This function prompts the user's connected wallet to sign a message and returns the signature.
const { openGlideDeposit } = useGlideDeposit({
...
walletProvider: {
...
signTypedDataAsync: async (data) => {
// Sign the message using the user's connected wallet
// Return the signature
},
},
});
Optional - Success callback
If you need to perform any actions after a successful deposit/withdrawal, you can pass a onSuccess
callback to the useGlideDeposit
hook.
const { openGlideDeposit } = useGlideDeposit({
...
onSuccess: (txHash) => {
// Perform actions after a successful deposit/withdrawal
},
});
Default Mode
If you want to open the deposital model with a specific mode, you can set the mode
property to either "withdraw" or "deposit". This will skip the mode selection screen and directly open the specified mode.
const { openGlideDeposit } = useGlideDeposit({
app: "my-app",
recipient: "0x0...",
/* Set the mode to "withdraw" or "deposit" */
mode: "withdraw" | "deposit",
walletProvider: { ... },
});
You can now call the openGlideDeposit
function to open the withdraw modal.
<button onClick={() => openGlideDeposit()}>Open</button>